
Welcome to the Kaia Docs
SDK Documentation
Build freely with tools tailored for your language.
Seamlessly integrate with Kaia Network using our enhanced SDKs for JavaScript, Java, Python and more. Built on trusted foundations like Ethers, Web3.js, Web3j, and Web3.py, our libraries give you the power to submit transactions, read smart contracts, and develop complex applications with extended functionality - all in your preferred programming language.
View More SDKs →JSON-RPC API Reference
Discover and Engage with Kaia's JSON-RPC APIs
Unlock Kaia's full potential with our interactive API documentation. Test API calls directly in the docs, explore detailed request and response examples, and generate code snippets in curl, Python, Node.js, and Java. Whether developing new applications or integrating with existing systems, our comprehensive API reference provides the tools for efficient development on the Kaia platform.
Get started with Kaia's JSON RPC APIs →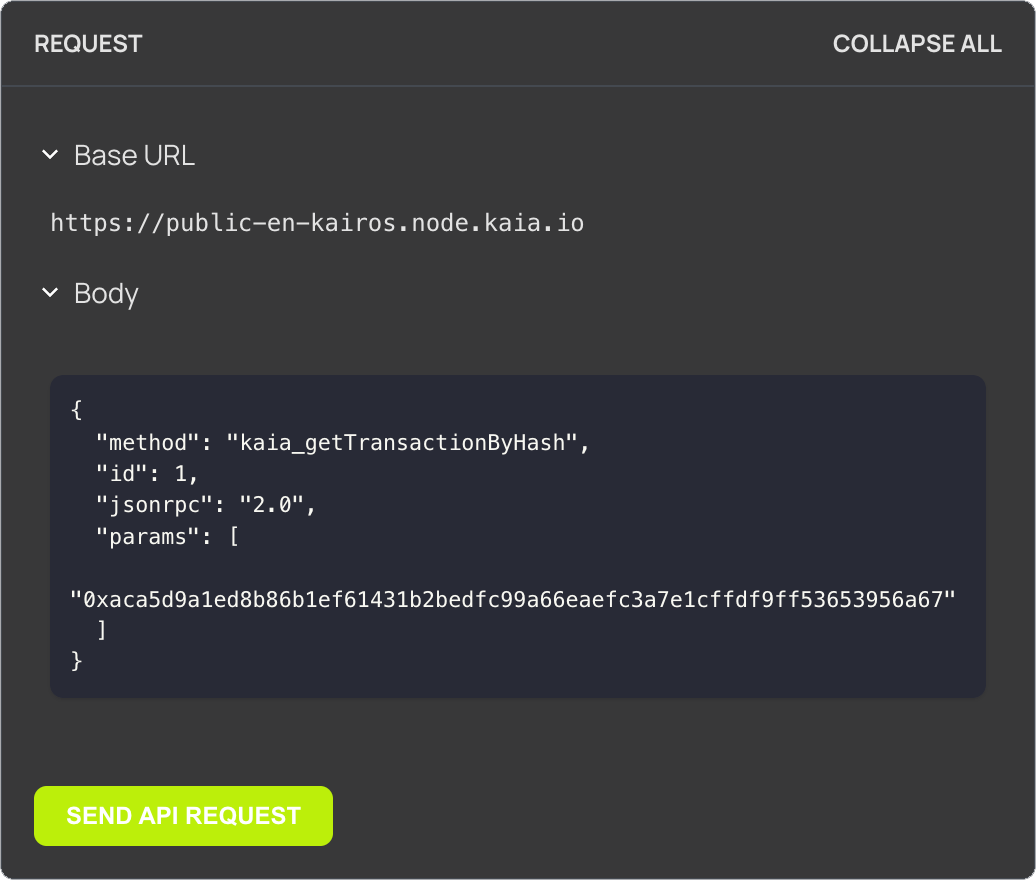