What's New
Explore our latest updates and additions to the docs.
Empower AI Agents on Kaia Blockchain!
Unlock seamless blockchain integration for your AI agents—enable secure, autonomous onchain actions and DeFi operations using Kaia Agent Kit with any AI framework.
Live Code Editor Now Available in SDK Docs!
Try out code instantly in Kaia Docs! The SDK pages now feature a live CodeSandbox editor for Ethers-ext (v6) and Web3js-ext Account Management.
Adding llms.txt for AI Bot Control
Implement llms.txt in your project to manage AI bot access to Kaia Docs.
SDK Documentation
Build freely with tools tailored for your language.
Seamlessly integrate with Kaia Network using our enhanced SDKs for JavaScript, Java, Python and more. Built on trusted foundations like Ethers, Web3.js, Web3j, and Web3.py, our libraries give you the power to submit transactions, read smart contracts, and develop complex applications with extended functionality - all in your preferred programming language.
View More SDKs →JSON-RPC API Reference
Discover and Engage with Kaia's JSON-RPC APIs
Unlock Kaia's full potential with our interactive API documentation. Test API calls directly in the docs, explore detailed request and response examples, and generate code snippets in curl, Python, Node.js, and Java. Whether developing new applications or integrating with existing systems, our comprehensive API reference provides the tools for efficient development on the Kaia platform.
Get started with Kaia's JSON RPC APIs →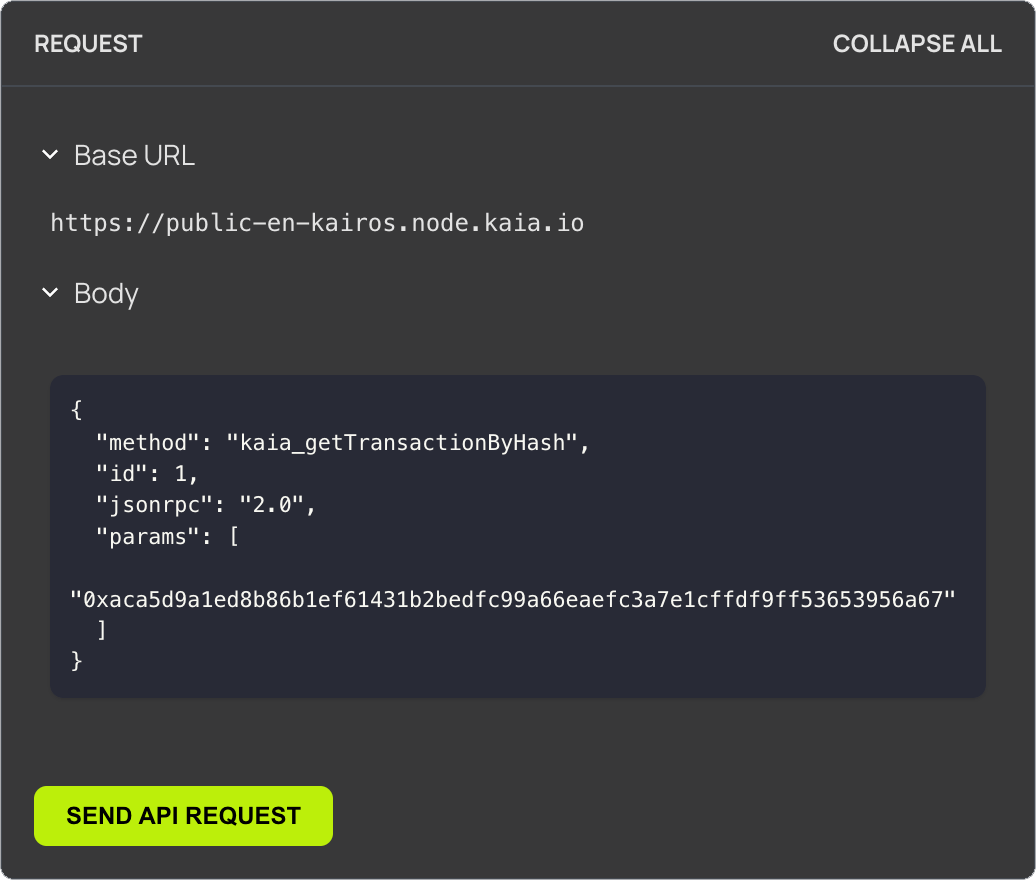